As part of my day job, I needed to answer a simple question: Who has access to a certain network shared drive and what level of access do they have i.e. read/write?
I hate capturing data manually. And also NOT in a form that will be amenable to analysis. I was just itching to automate this little process. I tried looking for something quick and easy in Python with no luck. If you are aware of something like that, please do let me know in the comments.
There was some reluctance on my part to learn PowerShell, even though I've done some scripting in it before, but I didn't want to invest time doing that. Eventually, I ended up doing the PowerShell script. It wasn't as bad as I thought it would be and it took less than 2 hours 🙂
There are two scenarios you might face when obtaining ACL (Access Control List) information about a network shared folder.
Scenario 1: You don't have access to the Network Folder you want to audit but can get a user who has access to the folder to run some PowerShell commands for you
In this case, you will run the following command to get the ACL for a network shared folder:
Get-Acl \\path.to.network\shared\folder | fl > my-choosen-file-name.txt
The output file looks something like the following highly redacted image :P
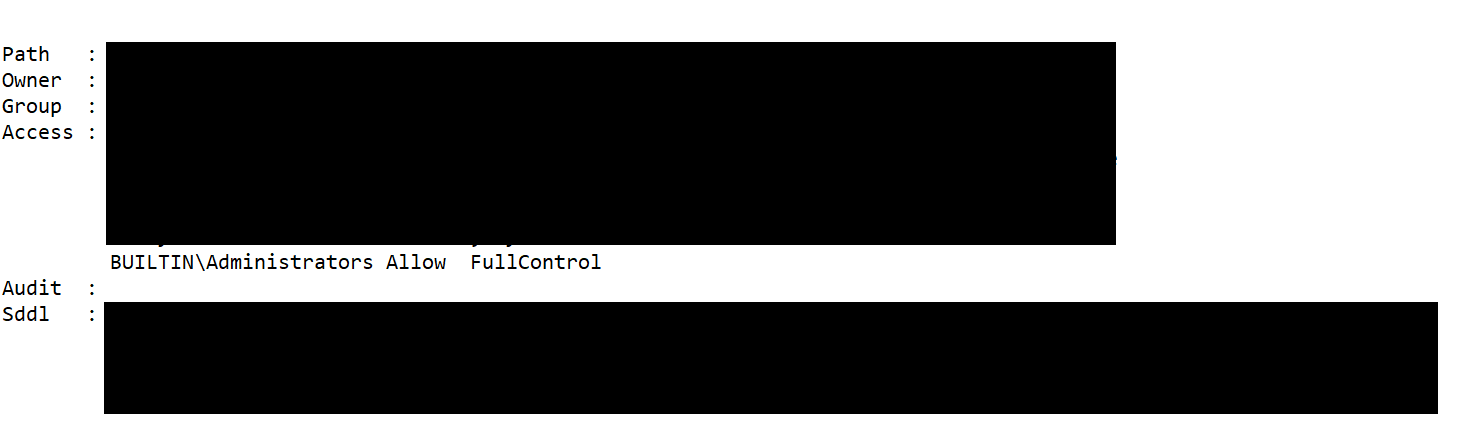
You will see groups and usernames that have access to the folder in the Access field.
Collect the group names and provide that as input to the following powershell script which you'll have to run in PowerShell (duh!).
function GetACLByFolders{
$inputGroups | ForEach-Object {
$inputGroup = $_
Get-ADGroupMember $inputGroup | Select SamAccountName, objectClass| ForEach-Object {
if($_.objectClass -eq "group") {
$currentGroup = $_.SamAccountName
Get-ADGroupMember $_.SamAccountName | Select SamAccountName, objectClass | ForEach-Object {
Get-ADUser -Identity $_.SamAccountName -Properties * | Select SamAccountName, Name, Title, City, co, Company, Country, Created, Deleted, LastLogonDate, LockedOut, Office, PasswordExpired
}
} else {
$currentGroup = $inputGroup
Get-ADUser -Identity $_.SamAccountName -Properties * | Select SamAccountName, Name, Title, City, co, Company, Country, Created, Deleted, LastLogonDate, LockedOut, Office, PasswordExpired
}
} | Select-Object @{Name="group";Expression={$currentGroup}},*
} | Sort-Object Created
}
#This is a list of group names
$inputGroups = @("Group 1","Group 2","Group 3")
#Use the following line if you just want to see the input on screen
#GetACLByFolders($inputGroups) | ft
#Use the following line if you want to export to csv
GetACLByFolders($inputGroups) | Export-CSV -Path 'MyOutputFileName.csv'
Scenario 2: You have read/list access to the folder you want to audit
In this case simply use the following script but put the path you want to audit as an input.
function GetACLByPath{
$ErrorActionPreference = 'SilentlyContinue'
Get-Acl $args[0] | Select-Object Access | ForEach-Object {
$_.Access | Select IdentityReference, AccessControlType, FileSystemRights } |
ForEach-Object {
#Note the following line may or maynot be neccessary in your case.
$inputGroup = $_.IdentityReference -replace "DOMAIN\\",""
$ACLType = $_.AccessControlType
$FileSystemRights = $_.FileSystemRights
Get-ADGroupMember $inputGroup | Select SamAccountName, objectClass| ForEach-Object {
if($_.objectClass -eq "group") {
$currentGroup = $_.SamAccountName
Get-ADGroupMember $_.SamAccountName | Select SamAccountName, objectClass | ForEach-Object {
#Configure AD attributes you may want to extract
Get-ADUser -Identity $_.SamAccountName -Properties * | Select SamAccountName, Name, Title, City, co, Company, Country, Created, Deleted, LastLogonDate, LockedOut, Office, PasswordExpired
}
} else {
$currentGroup = $inputGroup
Get-ADUser -Identity $_.SamAccountName -Properties * | Select SamAccountName, Name, Title, City, co, Company, Country, Created, Deleted, LastLogonDate, LockedOut, Office, PasswordExpired
}
} | Select-Object @{Name="group";Expression={$currentGroup}},@{Name="Type";Expression={$ACLType}},@{Name="Rights";Expression={$FileSystemRights}},*
} | Sort-Object Created
}
#This is the input folder location you need to change
$folder = "\\path.to.network\shared\folder"
#Use this for on screen output
GetACLByPath($folder) | ft
#Use this for csv output
GetACLByPath($folder) | Export-CSV -Path 'AccessToFolder.csv'
How to Audit Access to Windows Network Share Drives using Powershell Script
Use the power of PowerShell scripts to collect data about who has access to a Windows Network Share Drive.