I spin up multiple FastAPI projects a day to experiment with an idea I have. I have been using the same app structure and create it manually every time. I thought it was time to automate that.
Here is a bash script that creates a new FastAPI project with poetry.
#!/bin/bash
# check if the directory specified in the first argument exists
if [ -d "$1" ]; then
echo "Directory $1 already exists."
else
# create the directory if it does not exist
mkdir "$1"
fi
# cd into the specified directory
cd "$1"
# initialize a poetry project
poetry init -n
# add fastapi and uvicorn as dependencies
poetry add fastapi uvicorn
# create an app directory
mkdir app
# create an empty main.py file in the app directory
touch app/main.py
# populate main.py with a simple FastAPI app
cat << EOF > app/main.py
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def index():
return {"message": "Hello World"}
EOF
# Add the script to the pyproject.toml file
echo "
[tool.poetry.scripts]
server = 'uvicorn app.main:app --reload --port 8000'
" >> pyproject.toml
To use this script, save it to a file (e.g. create_app.sh
) and make it executable with the following command:
chmod +x create_app.sh
Then you can run the script like this:
./create_app.sh <directory-name>
Replace <directory-name>
with the name of the directory you want to create. The script will create the directory if it does not exist, initialize a poetry project in it, add fastapi and uvicorn as dependencies, create an app directory and a main.py file, and populate the main.py file with a simple FastAPI app.
Using this script to takes 5 seconds to setup a running FastAPI project:
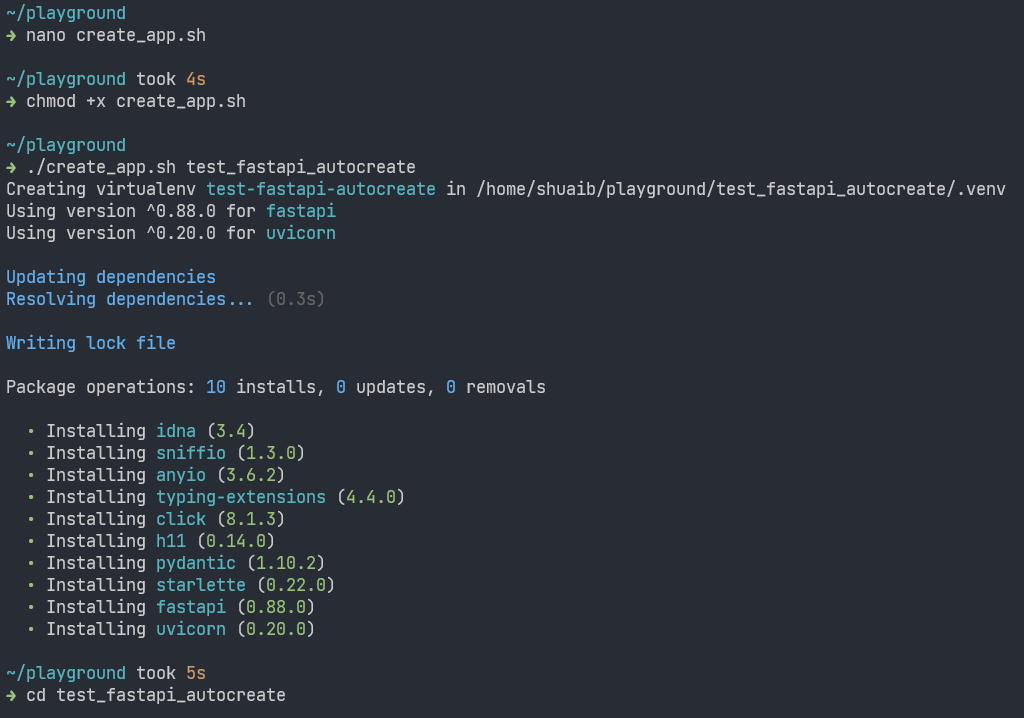